This page will help you get started with Maze.Guru API
Authentication Process
- Login in https://maze.guru/setting and get the App ID and App Key, Note: You must be a pro user
- After get the ID and Key, make sure to test the authentication process, the process is as below
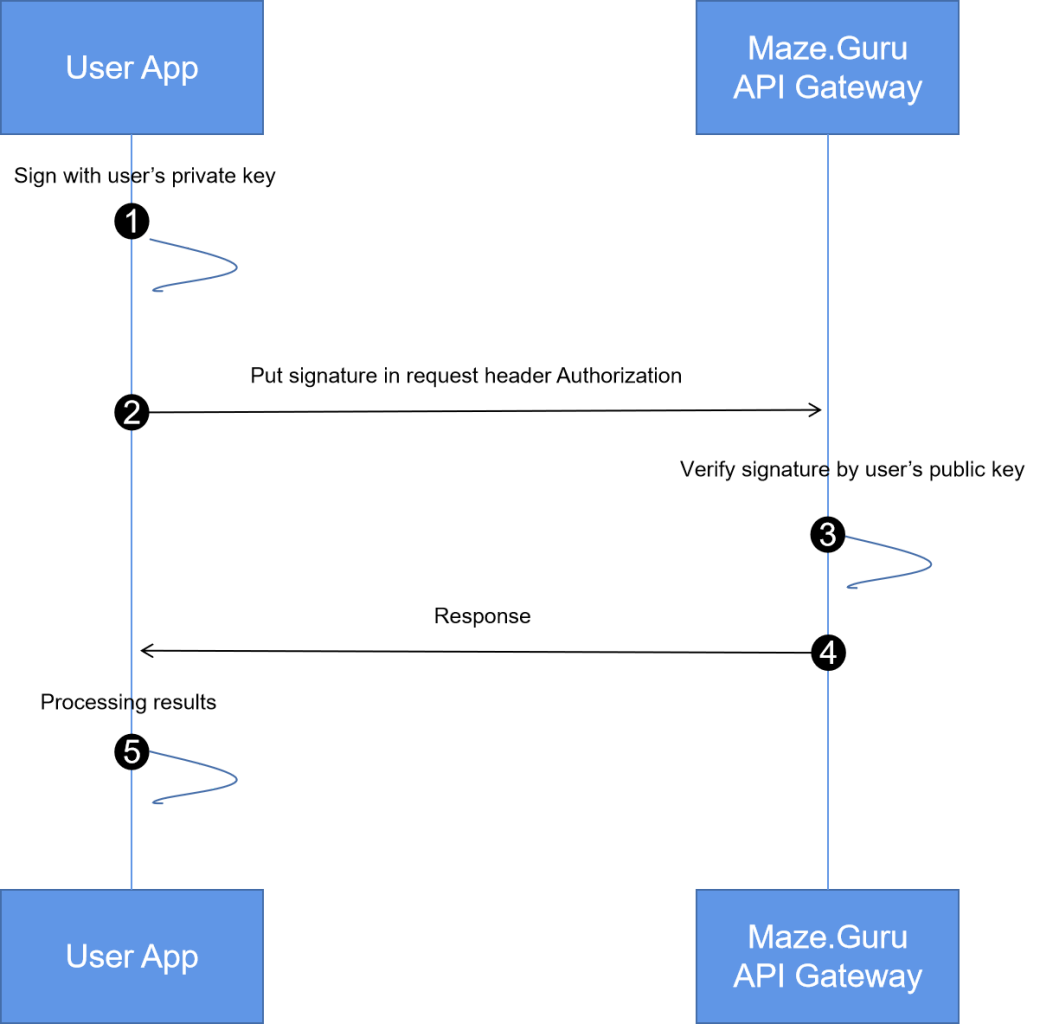
- Create signature original
{"appId":"appId","timestamp":xxxxx}
, use the private key for SHA256withRSA signature, and perform Base64 processing.(see the code demo below) - Add
Authorization
in the request header, and the value as{"secretKeyVersion":"1","appId":"appId","sign":"signature", "original": "signature original"}
- Make a HTTP request, the signature expiration time is in 5 minutes.
Code Demo
from Crypto.PublicKey import RSA
from Crypto.Hash import SHA256
from Crypto.Signature import pkcs1_15
import requests
import time
import json
import base64
# RSA Signature
def rsa_sign(data, rsa_key):
h = SHA256.new(data.encode('utf-8'))
signature = pkcs1_15.new(rsa_key).sign(h)
return str(base64.b64encode(signature), encoding = "utf-8")
# RSA Verification
def rsa_sign_verify(data, sig, rsa_key):
try:
h = SHA256.new(data.encode('utf-8'))
pkcs1_15.new(rsa_key).verify(h, base64.b64decode(sig))
ret = True
except (ValueError, TypeError):
ret = False
return ret
def test(appid):
now = int(time.time())
# build message
plain_text = {
"appId": appid,
"timestamp": now
}
original = json.dumps(plain_text, separators=(',', ':'))
print(f"original {original}")
auth = {
"appId": appid,
"secretKeyVersion": "1",
"sign": sign(original),
"original": original
}
headers = {"Authorization": json.dumps(auth, separators=(',', ':'))}
print(f"headers {headers}")
response = requests.get(f"https://open.maze.guru/api/v1/style-base-infos", headers=headers)
response.encoding = "utf-8"
def sign(text):
private_key_base64_str = 'MIICdgIBADANBgkqhkiG9w0BAQEFAASCAmAwggJcAgEAAoGBAKuaRcAYkmDxKObdC5YNGllsMCdnNNLE71zEmvScqAdNCBbf3gaytd5BtN9v+YdZYqXSq8WkyhfRRHGPZ/O7zQ/4I1MKK7wNTeyVmarwMfCkSxIa5coqJTV68dqWayI69pvQLYBqwfKqiMbvDY/WrvpA8uSRPSMfiJttyr7Ei/rVAgMBAAECgYA+R2IzInfVmRCYIPc4gQ7kD2C2nD1OlUyt5Wi7iDNvWuSpsJva4Hci78PeJ7xvA+DKf9f5hiIWXMuzTzGSAsGcBvY0rgghpBKfX58pL3Ete/cny4UGpBwuVWAgQo+HG4XywIti9KfWJaX8gzEdT/q81N5tOWJeVGdfECs+JDNRwQJBANJG7xdU+9XMZWmxXlm4W2WTDVGPMqPPqfy3oWiAa0Q9K5su0pIEeGEcobxF9CMWpuej5Ontxo2uM7T9h3ShFXECQQDQ6oa19wLnF5UIIAsTwNtgL2UIksQ+cEwSK/+cabkCGbCLHpuXjOUuOQIWVj73PZAAyknugF53bBbaXk9UozmlAkAFsSHbsdM3UFcWa1SwfQsMTtS3dnbadE6XpqjZ2VAOAtHhW30bsr9Hcaz3GbWoFX7jGk6h/mu3fhWsOoimIY4hAkAkWMk7G1CL+BRbp4eF1kXUhCplxXMTEeKKPtFn2xTvAp0udxLG2SJ+Wji8viCST0mgeZFHR1ooGMwZDdNrfEJpAkEApG6GgYbzqYSbxon2n2QuUif8j6XyOKpveQbLhjcCCy3BOGV1+JSYZ+iuueGBbHXnBxlfCXC8RJdhwpJjQRoV8Q=='
public_key_base64_str = 'MIGfMA0GCSqGSIb3DQEBAQUAA4GNADCBiQKBgQCrmkXAGJJg8Sjm3QuWDRpZbDAnZzTSxO9cxJr0nKgHTQgW394GsrXeQbTfb/mHWWKl0qvFpMoX0URxj2fzu80P+CNTCiu8DU3slZmq8DHwpEsSGuXKKiU1evHalmsiOvab0C2AasHyqojG7w2P1q76QPLkkT0jH4ibbcq+xIv61QIDAQAB'
private_key=base64.b64decode(private_key_base64_str)
public_key=base64.b64decode(public_key_base64_str)
sign = rsa_sign(text, RSA.importKey(private_key))
print(f"sign:{sign}")
sign_result = rsa_sign_verify(text, sign, RSA.importKey(public_key))
print(f"sign_result:{sign_result}")
return sign
if __name__ == "__main__":
appid = 'wja0q53lmql4cm2yd1'
test(appid)
Gateway URL(Base URL)
QPS limitation
Maze.guru sets qps to 50
Response format
Normal return
{
"code": 200,
"msg": "",
"data": {}
}
Error return
{
"code": 201,
"msg": "Signature has expired",
"data": null
}
Error code
Error code | Error message |
---|---|
20110001 | The prompt contains sensitive words |
20110002 | Incorrect or unsupported seed image link |
20110003 | Seed image contains sensitive information |
20110004 | Job does not exist |
20110005 | Job key does not exist |
20110007 | Model does not exist |
20110008 | The job is being generated and cannot be revoked/accelerated |
20110009 | The status of the job has changed, please refresh |
20110010 | Insufficient points balance |
20110011 | The job is not in the queue and cannot be revoked |
20010015 | Unsupported sizes/resolutions |
20110000 | Text translation failed |
1000 | err unknown |
1001 | Invalid request param |
1005 | Token authentication fail |
1024 | Invalid image param |
1054 | This artwork is high resolution |
1056 | style_id does not exist |
1062 | Signature expired |
1063 | The job not belong to current user |
1088 | Stylize must be between 0 and 1000 |
1090 | job not ready |